SUN dataset¶
This tutorial reads and visualizes an RGBDImage
of the SUN dataset [Song2015].
5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | # examples/Python/Basic/rgbd_sun.py
import open3d as o3d
import matplotlib.pyplot as plt
if __name__ == "__main__":
print("Read SUN dataset")
color_raw = o3d.io.read_image(
"../../TestData/RGBD/other_formats/SUN_color.jpg")
depth_raw = o3d.io.read_image(
"../../TestData/RGBD/other_formats/SUN_depth.png")
rgbd_image = o3d.geometry.RGBDImage.create_from_sun_format(
color_raw, depth_raw)
print(rgbd_image)
plt.subplot(1, 2, 1)
plt.title('SUN grayscale image')
plt.imshow(rgbd_image.color)
plt.subplot(1, 2, 2)
plt.title('SUN depth image')
plt.imshow(rgbd_image.depth)
plt.show()
pcd = o3d.geometry.PointCloud.create_from_rgbd_image(
rgbd_image,
o3d.camera.PinholeCameraIntrinsic(
o3d.camera.PinholeCameraIntrinsicParameters.PrimeSenseDefault))
# Flip it, otherwise the pointcloud will be upside down
pcd.transform([[1, 0, 0, 0], [0, -1, 0, 0], [0, 0, -1, 0], [0, 0, 0, 1]])
o3d.visualization.draw_geometries([pcd])
|
This tutorial is almost the same as the tutorial processing Redwood dataset. The only difference is that we use conversion function create_rgbd_image_from_sun_format
to parse depth images in the SUN dataset.
Similarly, the RGBDImage
can be rendered as numpy arrays:
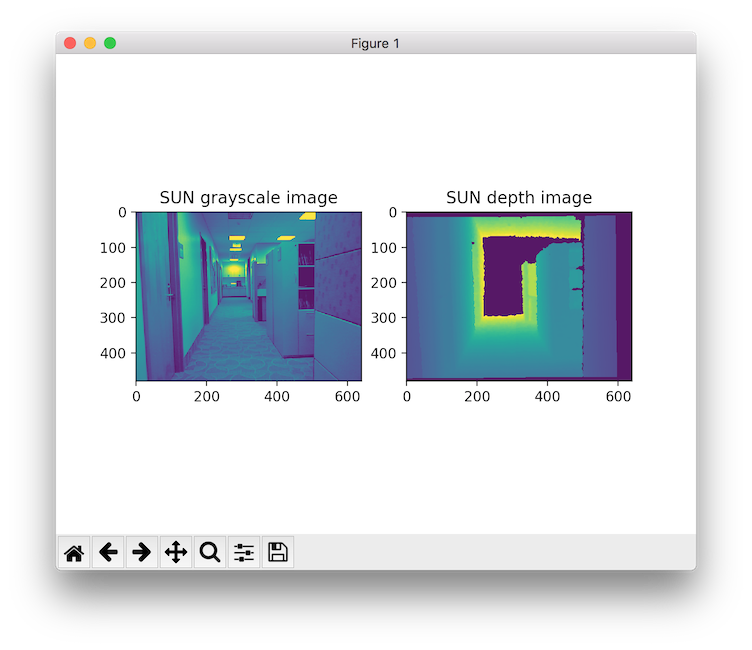
Or a point cloud:
